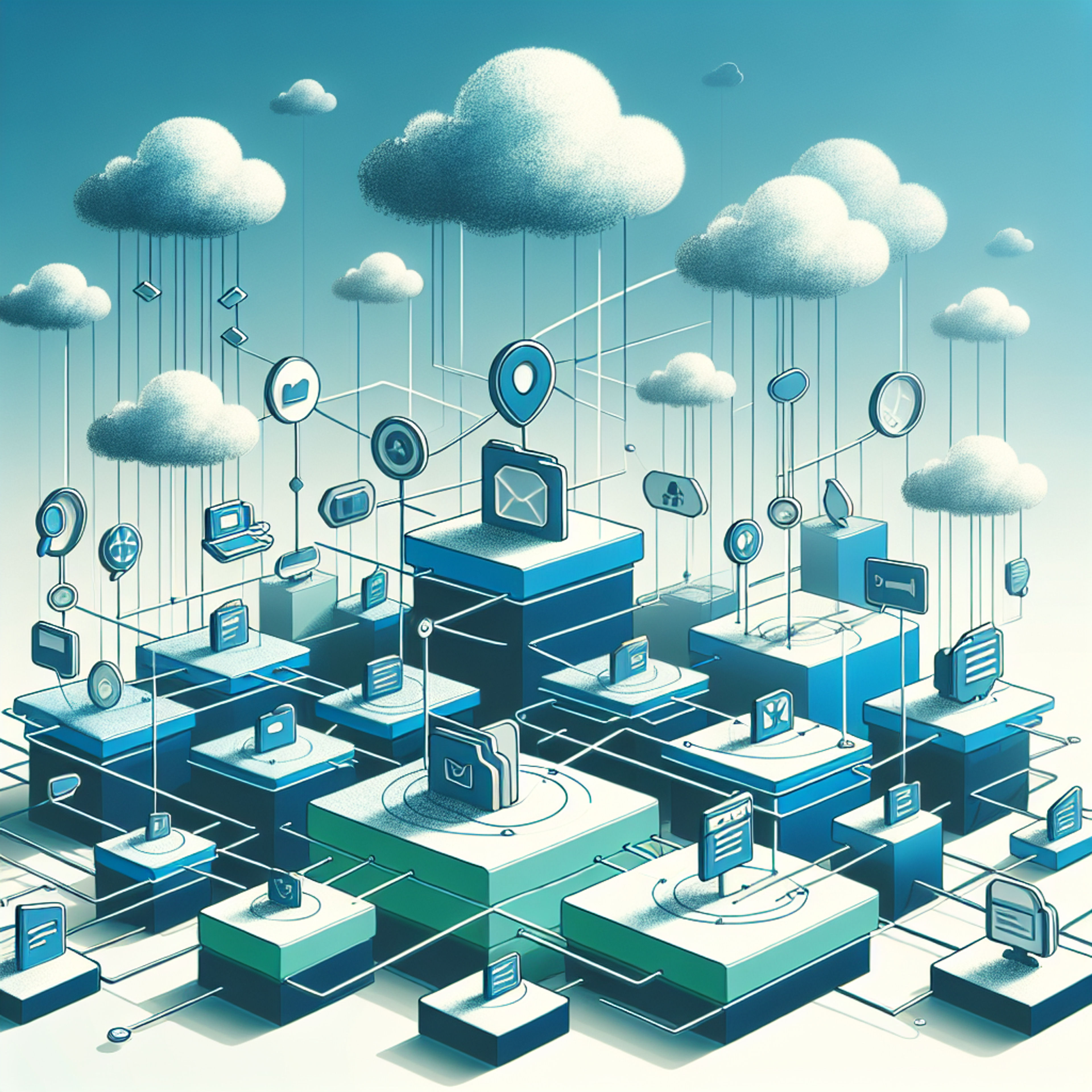
A sitemap is a file that lists all the pages on a website and provides information about their organization and structure.
It helps search engines understand the content and structure of a website, making it easier for them to crawl and index the site.
In this post, we’ll go through various methods to create an XML Sitemap in your Next.js project.
What is a Sitemap?
A sitemap is a file that contains a list of URLs on a website. It helps search engines discover and understand the structure of a website, making it easier for them to crawl and index the site.
There are 2 types of sitemaps:
-
XML sitemaps: Sitemaps are written in a specific format designed for search engines to crawl
-
HTML sitemaps: Sitemaps that look like regular pages that help users navigate the website
XML Sitemaps include additional information about each URL, such as the last modified date and the frequency of changes that make it easy for search engines to easily detect new content and crawl your website more efficiently.
Why Do You Need a Sitemap for Your Website?
When you set up Google Search Console for your website, you need to make sure you submit an XML sitemap.
A sitemap helps search engines crawl and index your website more efficiently. By providing a clearly defined sitemap, you can ensure that search engines can easily find and understand your website structure and its content.
This can improve your website's visibility in search engine results and ultimately drive more organic traffic to your site.
Organic traffic is the main traffic for my first side project, OpenGraph.xyz, which I sold for 5 figures.
How to Create Sitemap in a Next.js App Router Project
Next.js version 13.3 and above supports file-based metadata convention. Each file convention can be defined using a static file (e.g sitemap.xml
) or generated dynamically using code (e.g sitemap.(js|ts)
).
Once a file is defined, Next.js will automatically serve the file (with hashes in production for caching) and update the relevant head elements with the correct metadata.
1. Static XML Sitemap File
If you have a relatively simple and static website, you could manually create a sitemap.xml
file in the root of your app
directory of your project.
First, create a new file in the app
directory of your Next.js project and name it sitemap.xml
.
Next, open the app/sitemap.xml
file in a text editor and add the necessary XML structure.
app/sitemap.xml
<urlset xmlns="http://www.sitemaps.org/schemas/sitemap/0.9">
<url>
<loc>https://acme.com</loc>
<lastmod>2023-04-06T15:02:24.021Z</lastmod>
<changefreq>yearly</changefreq>
<priority>1</priority>
</url>
<url>
<loc>https://acme.com/about</loc>
<lastmod>2023-04-06T15:02:24.021Z</lastmod>
<changefreq>monthly</changefreq>
<priority>0.8</priority>
</url>
<url>
<loc>https://acme.com/blog</loc>
<lastmod>2023-04-06T15:02:24.021Z</lastmod>
<changefreq>weekly</changefreq>
<priority>0.5</priority>
</url>
</urlset>
2. Generate Dynamic Sitemap using Code
Dynamic URLs are the pages on your website that are generated dynamically based on user input or other factors. These can include product pages, blog posts, or search result pages.
To add dynamic URLs to your sitemap, you can use the sitemap.(js|ts)
file convention to programmatically generate a sitemap by exporting a default function that returns an array of URLs. If using TypeScript, a Sitemap
type is available.
Dynamic Sitemap using Code
import { MetadataRoute } from 'next'
export default function sitemap(): MetadataRoute.Sitemap {
const res = await fetch('https://.../posts');
const allPosts = await res.json();
const posts = allPosts.map((post) => ({
url: `https://acme.com/blog/${post.slug}`,
lastModified: post.publishedAt,
}));
const routes = ['', '/about', '/blog'].map((route) => ({
url: `https://acme.com${route}`,
lastModified: new Date().toISOString(),
}));
return [...routes, ...posts];
}
Breaking down your sitemaps
Sitemaps have a limit of 50K URLs per file. If your website has tons of pages, it’s recommended to break it down into smaller sitemaps that make it easier to maintain.
In Next.js, there are two ways you can create multiple sitemaps:
-
By nesting
sitemap.(xml|js|ts)
inside multiple route segments e.g.app/sitemap.xml
andapp/products/sitemap.xml
. -
By using the
generateSitemaps
function.
For example, to split a sitemap with generateSitemaps
, return an array of objects with the sitemap id
. Then, use them to generate unique sitemaps.
sitemap.(xml|js|ts)
import { BASE_URL } from '@/app/lib/constants'
export async function generateSitemaps() {
// Fetch the total number of products and calculate the number of sitemaps needed
return [{ id: 0 }, { id: 1 }, { id: 2 }, { id: 3 }]
}
export default async function sitemap({
id,
}: {
id: number
}): Promise<MetadataRoute.Sitemap> {
// Google's limit is 50,000 URLs per sitemap
const start = id * 50000
const end = start + 50000
const products = await getProducts(
`SELECT id, date FROM products WHERE id BETWEEN ${start} AND ${end}`
)
return products.map((product) => ({
url: `${BASE_URL}/product/${id}`,
lastModified: product.date,
}))
}
3. Use Next-Sitemap
Next-sitemap is a sitemap generator for Next.js. It generates sitemaps for all static/pre-rendered/dynamic/server-side pages.
Next-sitemap is easy to use and I’ve been using it on all my Next.js projects. This is a sample of sitemap for Yuurrific.com.
First, create a basic config file, next-sitemap.config.js
, in the root of your app
directory of your project.
next-sitemap.config.js
module.exports = {
siteUrl: process.env.SITE_URL || 'https://example.com',
generateRobotsTxt: true, // (optional)
// ...other options
}
Next, add the command to your postbuild
script in package.json
.
Update package.json
"scripts": {
"dev": "next dev",
"turbo": "next dev --turbo",
"build": "next build",
"start": "next start",
"lint": "next lint",
"preview": "next build && next start",
"postbuild": "next-sitemap --config next-sitemap.config.js"
},
With Next-sitemap, it’s easy to split large sitemap into multiple sitemaps by defining the sitemapSize
property in the config.
Define sitemap size
module.exports = {
siteUrl: 'https://example.com',
generateRobotsTxt: true,
sitemapSize: 5000,
}
By setting sitemapSize
to 5000
, next-sitemap
would create an index sitemap and the other sitemap files once the number of URLs hits more than 5000 URLs.
It will output the following files:
-
sitemap.xml
-
sitemap-0.xml
-
sitemap-1.xml
, etc
next-sitemap
also has a transformation
function you could use if you rewrite URLs via middleware as that would affect how the URLs are generated in the sitemaps.
Submitting the Sitemap to Search Engines
Once you have created and generated your sitemap in Next.js, it's important to submit it to search engines to ensure they crawl and index your website effectively.
Google Search Console
Google Search Console is a free tool provided by Google that allows you to monitor and optimize your website's presence in Google search results.
You can submit your sitemap to Google Search Console to ensure that Google crawls and indexes your website correctly.
Bing Webmaster Tools
Bing Webmaster Tools is a similar tool provided by Bing, the second-largest search engine. You can submit your sitemap to Bing Webmaster Tools to ensure that Bing crawls and indexes your website effectively.
Conclusion
In conclusion, creating a sitemap in Next.JS is crucial for optimizing your website for search engines and improving its visibility.
By following the step-by-step guide outlined above, you can easily create and generate a sitemap in Next.JS.
Remember to submit your sitemap to search engines like Google and Bing to ensure they crawl and index your website effectively.